CPP Assignments
- C# Two Dimensional String Array
- One Dimensional And Two Dimensional Array In C In C++ Example With Output
- One Dimensional And Two Dimensional Array In C++
- How One Dimensional And Two Dimensional Array Are Created In Foxpro
CPP Assignment for our Cpp Tutorials for Beginners
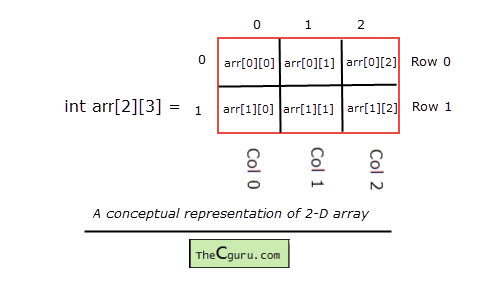
- Double Dimensional Array – Assignment
- Single Dimensional Array – Assignment-1
- String in CPP Assignment-1
- String Handling in Cpp with Assignment
- Two Dimensional Arrays in C++ with Assignment
- Communication and Networking Concept Notes – Class 12
- File handling in C++ Assignment
- Class 12 Computer Science Practical File download
- Class 11 computer science assignment
- Class 12 Computer Science SQL Notes
- C++ flow of control assignment
In C++ Two Dimensional array in C++ is an array that consists of more than one rows and more than one column. In 2-D array, each element is referred by two indexes. Elements stored in these Arrays in the form of matrices. The first index shows a row of the matrix and the second index shows the column of the matrix.
Two Dimensional Array in C The Two Dimensional Array in C language is nothing but an Array of Arrays. If the data is linear, we can use the One Dimensional Array. However, to work with multi-level data, we have to use the Multi-Dimensional Array. An Array having more than one dimension is called Multi Dimensional array in C. In our previous article, we discussed Two Dimensional Array, which is the simplest form of C Multi Dimensional Array. In C Programming Language, by placing n number of brackets , we can declare n-dimensional array where n is dimension number. The memory for the pointer array and the two-dimensional array elements is usually allocated separately using dynamic memory allocation functions, namely, malloc or calloc. A simplified function imatalloc given below allocates a regular integer matrix of size m x n to variable tmp of type int. and returns this pointer to the calling function.
The syntax of Two-Dimensional Array:-
Data type ArrayName [Number of rows] [Number of columns];
Int x[3] 47];
When we type the above statement the compiler will generate a 2-D array of a matrix which consists of 3 rows and 4 columns.
Accessing each location of Two Dimensional Arrays:-
In order to store values in a C++ two-dimensional array, the programmer has to specify the number of rows and the number of column of that array.
To access each individual location of a matrix to store the values the user has to provide the exact number of row and number of column.
For Example:-
int matrix [2][2];
matrix [0] [0] = 43;
matrix [0] [1] = 44;
matrix [1] [0] = 56;
matrix [1] [1] = 72;
How to enter data in a Two Dimensional Arrays/ Array initialization methods
The 2-D arrays which are declared by the keyword int are able to store integer values are called two-dimensional integer arrays. The process of assigning values during declaration is called initialization. These Arrays can be initialized by putting the curly braces around each row separating by a comma also each element of a matrix should be separated by a comma.
1st Method
int x[2][3] = {10,20,30,40,50,60 };
IN this case, your compiler will automatically locate the first three values in the first row and the last 3 values in the next row.
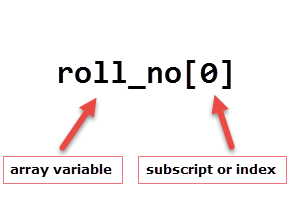
2nd method :
int x[2][3]= { {10,20,30},{40,50,60}};
now, in this case, the value of the first row is clear as well as the value in the second row.
3rd Method:
int x[2][3];
C# Two Dimensional String Array
x[0][0]=10;
x[0][1]=20;
x[0][2]=30;
x[1][0]=40;
x[1][1]=50;
x[1][2]=60;
IN the above example values has been assigned using accessing individual location.
4th method:
int x[2][3];
cin>>x[0][0]>>x[0][1]……………………………………………>>x[1][2];
in this case, the value of that array has been populated at run time.
5th Method:
For Example:-
#include<iostream>
int main(){
int matrix [5] [5];
for (int m1=0 ; m1<5 ; m1++){
for (int m2=0 ; m2<5 ; m2++){
matrix [m1] [m2] = 125 ;
}
}
return 0;
}
The above portion of code uses nested F loop to assigns 5 to all the locations of a matrix of a two-dimensional array in C++.
6th Method: Only allowed when you declare as well as initialize your array at the same time. Example of this method is as follows
int x[][3] ={10,20,30,40,50,60};
in this case, your compiler will automatically generate a total of two rows as it will divide total elements with three and result will be its rows.
Do not Try to initialize these type of array
Double Dimensional Array initialization Mistake Example: -1
int x[2][] ={ 10,20,30,40,50,60};
as in this case, your system will not be able to calculate the total number of columns and will result in a syntax error.
Double Dimensional Array initialization Mistake Example: -2
int x[][3];
The blank declaration is not allowed.
Double Dimensional Array initialization Mistake Example : -3
int x[n][3];
Not allowed as ‘n’ is not defined.
Output Method for Double Dimensional Array
Since double dimensional array is most of the time used for solving matrix type problems thus sometimes programmer call it ‘matrix’ so they love to display double dimensional array in a tabular format.
1st Method to Display Two dimensional Array in Tabular format
int x[2][3] = {10,20,30,40,50,60}
for (int I =0;i<2;i++ )
for(int j=0;j<3;j++)
cout<<setw(6)<<x[i][j];
cout<<endl;
}
2nd Method to Display Two dimensional Array in Tabular format
int x[2][3] = {10,20,30,40,50,60}
for (int I =0;i<2;i++ ){
cout<<endl;
for(int j=0;j<3;j++)
cout<<setw(6)<<x[i][j];
}
C++ Program to read a double dimensional array integer of order 3×4 . Find out the sum of element and then display entered array as well as sum of these elements on the screen.
#include<iostream>
#include<iomanip>
using namespace std;
int main(){
int x[2][3],i,j,sum;
//input phase
for(i=0;i<2;i++)
for(j=0;j<3;j++){
cout<<“Enter x[“<<i<<“][“<<j<<“] Element :”;
cin>>x[i][j];
}
//processing phase
sum =0;
for(i=0;i<2;i++)
for(j=0;j<3;j++)
sum+=x[i][j] ;
//output phase
cout<<“nn Sum of elements :”<<sum;
for(i=0;i<2;i++)
{
cout<<endl;
for(j=0;j<3;j++)

cout<<setw(6)<<x[i][j];
}
return 0;
}
Sample Output
C++ Assignment on Two Dimensional Array
Q1. Write a program in C++ to read an array of the integer of order 3×4. Find out the sum of only those elements which is either divisible by 3 or 7. Display sum of these elements and entered array in tabular format on the screen.
Q2. Write a program in C++ to read an array of an integer of order 3×4. Find out the sum of each row. Display sum each row as well as the entered array in tabular format on the screen.
Q3. Write a program in C++ to read an array of an integer of order 3×4. Find out the sum of each column. Display sum each column as well as entered array in tabular format on the screen.
Q4. Write a program in C++ to read an array of an integer of order 3×4. Interchange the elements of the first row with the last row of this array. Display this newly created array in tabular format on the screen.
Q5. Write a program in C++ to read an array of an integer of order 3×4. Give an increment of 20 to each element of this array. Display this modified array on the screen in a tabular format.
Q6. Write a program in C++ to read an array of integers of size 4×4. Display lower triangular matrix of this matrix on the screen.
Q7. Write a program in C++ to read an array of integers of size 4×4. Display upper triangular matrix of this matrix on the screen.
One Dimensional And Two Dimensional Array In C In C++ Example With Output
Q8. Write a program in C++ to read an array of integers of size 4×4. Display diagonal matrix of this matrix on the screen.
Q9 Write a program in C++ to read a matrix of integers of size 4×4. Find out Transpose of this matrix and display this newly created Transpose matrix on the screen.
Q10. Write a program in C++ to read two matrices A and B of order 3×4. Find out the Addition of these two matrices. Display Both entered matrix as well the sum of these two matrices on the screen.
Q11. Write a program in C++ to read two matrices A and B of order 3×4. Find out the subtraction of these two matrices. Display Both entered matrix as well the sum of these two matrices on the screen.
Related Posts
- Operators and Expressions in C++
- Flow of Control in C++
- Functions in C++
- Structure in CPP Notes in PDF format
- Class – Object – C plus plus Notes in PDF format
- Bubble sort in Single dimensional array
- Selection sort method in Single dimensional array
- Constructor Destructor in C++ Notes – PDF format
- Inheritance in C++
- Linear Search Program using 1-D Array
- File handling in C++ for CBSE class 12th student
- C++ File handling Notes in PDF Format
- Link list implemented Stack
- Basic Operation on Text File in C++
- Double Dimensional Array – Assignment
- String in CPP Assignment-1
- String in Cpp- Assignment No-1
- CPP program to Edit a record in Binary file
- CPP program to delete a record from a binary file
- Single Dimensional Array in CPP
One Dimensional And Two Dimensional Array In C++
- CPP
- Double Dimensional Array
- Double Dimensional Array Assignment
How One Dimensional And Two Dimensional Array Are Created In Foxpro
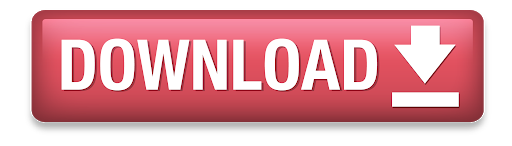